Second Practice Quiz¶
CS20-CP1 Apply various problem-solving strategies to solve programming problems throughout Computer Science 20.
CS20-CP2 Use common coding techniques to enhance code elegance and troubleshoot errors throughout Computer Science 20.
CS20-FP2 Investigate how control structures affect program flow.
CS20-FP3 Construct and utilize functions to create reusable pieces of code.
To confirm that you understand the major concepts you’ve seen with Reeborg, try to answer the first 4 questions without opening the Reeborg environment.
Question 1 - While Loops¶
- east
- Try again!
- south
- Try again!
- west
- Try again!
- north
- Great!
reeborg-second-quiz1: Reeborg is facing east. The following code is then executed:
while not is_facing_north():
turn_left()
What direction is the robot is facing now?
Question 2 - While and Repeat Loops¶
- east
- Try again!
- south
- Try again!
- west
- Great!
- north
- Try again!
reeborg-second-quiz2: Reeborg is facing east. The following code is then executed:
while not is_facing_north():
turn_left()
repeat 5:
turn_left()
What direction is the robot is facing now?
Question 3 - Loops and If¶
reeborg-second-quiz3: Reeborg is standing in a world in which every location square has 3 carrots, which looks like this:

The following code is then executed:
repeat 8:
if object_here():
repeat 2:
take()
move()
How many carrots is Reeborg holding now?
If you have spent time tracing this code on your own, and still cannot come up with the correct solution, you may find it helpful to open this world in the Reeborg environment. You can copy/paste the code above into the world, and step through the code one line at a time.
Question 4 - Loops and If/Else¶
reeborg-second-quiz4: Reeborg is holding a large handful of carrots, and is planning to plant them as it walks around a world that looks like this:
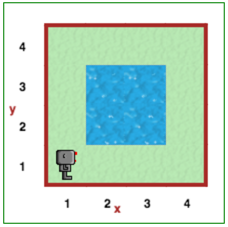
The following code is then executed:
repeat 7:
if front_is_clear():
move()
else:
turn_left()
put()
How many carrots has Reeborg planted when the code has finished?
If you have spent time tracing this code on your own, and still cannot come up with the correct solution, you may find it helpful to open this world in the Reeborg environment. You can copy/paste the code above into the world, and step through the code one line at a time.
Question 5 - Mirror Image¶
The room (shown below) has alcoves on the left and the right. Some of the alcoves contain daisies. For each alcove on the left side that contains a daisy, make Reeborg move the daisy to the opposite alcove on the right hand side. One possible starting world could look like the following:
Open the Mirror Image Practice Quiz world and create a solution to this problem!
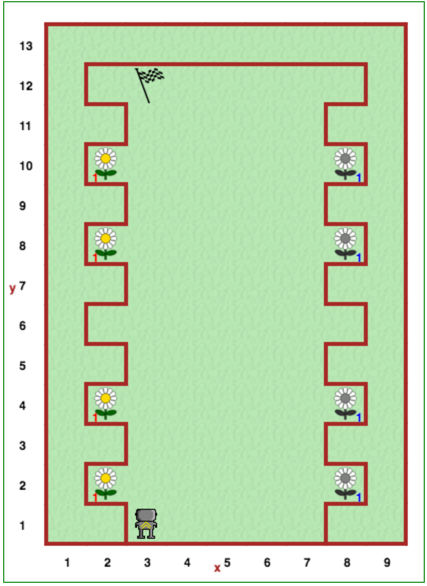
Do not look at this sample solution unless you have already finished creating your own solution!
Since all of the distances in the world stay exactly the same each time, this problem can be solved using only repeat loops. Please note that there are many possible solutions to this problem. This is one:
think(0)
def turn_around():
repeat 2:
turn_left()
def turn_right():
repeat 3:
turn_left()
def move_daisy():
take()
turn_around()
repeat 6:
move()
put()
turn_around()
repeat 5:
move()
turn_right()
repeat 6:
move()
turn_left()
move()
if object_here():
move_daisy()
else:
turn_around()
move()
turn_left()
if front_is_clear():
move()
Question 6 - Mirror Image - Part 2¶
This time, the distance between the alcove on the right and left is not consistent (in other words, the alcoves can be different distances apart). Once again, for each alcove on the left side that contains a daisy, make Reeborg move the daisy to the opposite alcove on the right hand side. One possible starting world could look like the following:
Open the Mirror Image Practice Quiz world and create a solution to this problem!
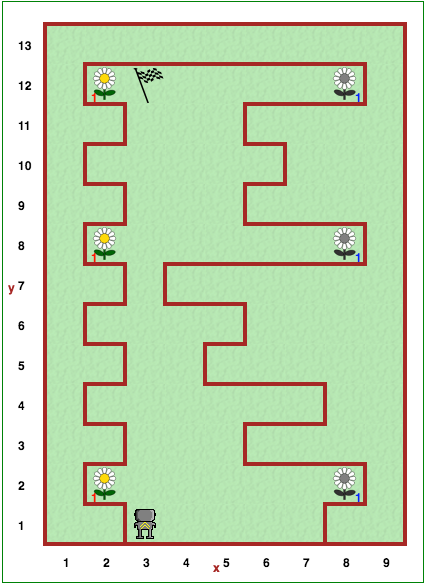
Do not look at this sample solution unless you have already finished creating your own solution!
Since there is an unknown distance to travel, you will need to use a while loop, instead of just a repeat loop. This is one possible solution:
think(0)
def turn_around():
repeat 2:
turn_left()
def turn_right():
repeat 3:
turn_left()
def backup():
turn_around()
move()
turn_around()
def move_daisy():
take()
turn_around()
while front_is_clear():
move()
put()
turn_around()
while front_is_clear():
move()
backup()
turn_right()
repeat 6:
move()
turn_left()
move()
if object_here():
move_daisy()
else:
turn_around()
move()
turn_left()
if front_is_clear():
move()