2. Python Review¶
2.1. Variables and Data Types¶
- boolean
- It is not True or False.
- integer
- The data is not numeric.
- float
- The value is not numeric with a decimal point.
- string
- Great! Strings are enclosed in quotes.
python-review1: What is the data type of 'what is your name?'
?
- boolean
- It is not True or False.
- integer
- Great! The data is numeric, without a decimal point.
- float
- The value is not numeric with a decimal point.
- string
- Strings are enclosed in quotes.
python-review2: What is the data type of 42
?
- boolean
- Great! Boolean is either True or False.
- integer
- The data is not numeric.
- float
- The value is not numeric with a decimal point.
- string
- Strings are enclosed in quotes.
python-review3: What is the data type of False
?
- boolean
- It is not True or False.
- integer
- The data is not numeric.
- float
- Great! The value is numeric with a decimal point.
- string
- Strings are enclosed in quotes.
python-review4: What is the data type of 4.5
?
2.2. Converting Between Data Types¶
Construct a block of code that correctly takes in a number from a user, then prints out twice the value that was given.
The following example is not going to work when you try to run it. Try entering in 16
, then 15
. Notice that nothing is printed either time, even though it looks like the conditional should be causing it to print. Can you figure out what is wrong and fix it? Hint: think about data types!
2.3. Math Operators¶
If you have trouble with any of the following, you might want to look back at the Math Operators list.
python-review7: What would the following code print?:
number = 12
print(number / 4)
python-review8: What would the following code print?:
number = 12
print(number % 5)
python-review9: What would the following code print?:
number = 42
print(number // 5)
python-review10: What would the following code print?:
number = 2
print(number ** 4)
python-review11: What would the following code print?:
number = 2
number += 4
print(number)
python-review12: What would the following code print?:
number = 9
number -= 2
print(number)
2.4. if
¶
Note
A single equal sign =
is used to assign a value. Two equal signs ==
are used when comparing a value.
- Output a
- Because -10 is less than 0, Python will execute the body of the if-statement here.
- Output b
- Python executes the body of the if-block as well as the statement that follows the if-block.
- Output c
- Python will also execute the statement that follows the if-block (because it is not enclosed in an else-block, but rather just a normal statement).
- It will cause an error because every if must have an else clause.
- It is valid to have an if-block without a corresponding else-block (though you cannot have an else-block without a corresponding if-block).
python-review13: What does the following code print?
x = -10
if x < 0:
print("The negative number ", x, " is not valid here.")
print("This is always printed")
a.
This is always printed
b.
The negative number -10 is not valid here
This is always printed
c.
The negative number -10 is not valid here
- Output a
- Try again. Remember that any number of consecutive if statements can evaluate to True.
- Output b
- Try again. Remember that any number of consecutive if statements can evaluate to True.
- Output c
- Try again. It's less than 100!
- Output d
- Great!
- It will cause an error because every if must have an else clause.
- Try again. An if statement does not require an else!
python-review14: What does the following code print?
number = 64
if number > 0:
print("It's positive!")
if number > 50:
print("It's pretty big.")
if number > 100:
print("It's really big!")
a.
It's positive!
b.
It's positive!
It's really big!
c.
It's pretty big.
d.
It's positive!
It's pretty big.
2.5. if/elif/else
¶
- Output 1
- TRUE is printed by the if-block, which only executes if the conditional (in this case, 4+5 == 10) is true. In this case 5+4 is not equal to 10.
- Output 2
- Since 4+5==10 evaluates to False, Python will skip over the if block and execute the statement in the else block.
- Output 3
- Python would never print both TRUE and FALSE because it will only execute one of the if-block or the else-block, but not both.
- Output 4
- Python will always execute either the if-block (if the condition is true) or the else-block (if the condition is false). It would never skip over both blocks.
python-review15: What does the following code print (choose from output a, b, c or nothing)?
if 4 + 5 == 10:
print("TRUE")
else:
print("FALSE")
a. TRUE
b. FALSE
c. TRUE
FALSE
d. Nothing will be printed.
- Output a
- Although TRUE is printed after the if-else statement completes, both blocks within the if-else statement print something too. In this case, Python would have had to have skipped both blocks in the if-else statement, which it never would do.
- Output b
- Because there is a TRUE printed after the if-else statement ends, Python will always print TRUE as the last statement.
- Output c
- Python will print FALSE from within the else-block (because 5+4 does not equal 10), and then print TRUE after the if-else statement completes.
- Output d
- To print these three lines, Python would have to execute both blocks in the if-else statement, which it can never do.
python-review16: What does the following code print?
if 4 + 5 == 10:
print("TRUE")
else:
print("FALSE")
print("TRUE")
a. TRUE
b.
TRUE
FALSE
c.
FALSE
TRUE
d.
TRUE
FALSE
TRUE
- Output a
- Great! Remember that only one branch of an if/elif/else block can execute.
- Output b
- Try again. Remember that only one branch of an if/elif/else block can execute.
- Output c
- Try again. Remember that only one branch of an if/elif/else block can execute.
- Output d
- Try again. Remember that only one branch of an if/elif/else block can execute.
python-review17: What does the following code print?
number = 64
if number > 0:
print("It's positive!")
elif number > 50:
print("It's pretty big.")
elif number > 100:
print("It's really big!")
a.
It's positive!
b.
It's positive!
It's really big!
c.
It's pretty big.
d.
It's positive!
It's pretty big.
2.6. while
loop¶
Construct a block of code that correctly counts from 10 down to 1, then prints Blastoff!
Write a program that asks the user to enter a password. Keep asking for the password until they enter “sask”. Once they have successfully typed in “sask”, print out What a great place!.
2.7. for
loop¶
Construct a block of code that correctly counts from 1 up to 10, then prints Made it!
Construct a block of code that correctly prints out a greeting for each person, using a for loop.
- 1
- The loop body prints one line, but the body will execute exactly one time for each element in the list [5, 4, 3, 2, 1, 0].
- 5
- Although the biggest number in the list is 5, there are actually 6 elements in the list.
- 6
- The loop body will execute (and print one line) for each of the 6 elements in the list [5, 4, 3, 2, 1, 0].
- 10
- The loop body will not execute more times than the number of elements in the list.
python-review22: In the following code, how many lines does this code print?
for number in [5, 4, 3, 2, 1, 0]:
print("I have", number, "cookies. I'm going to eat one.")
2.8. and
, or
, not
¶
- True
- Great!
- False
- Try again!
python-review23: What would the following print?:
a = 6
b = 10
print(a == 6)
- True
- Great!
- False
- Try again!
python-review24: What would the following print?:
a = 6
b = 10
print( not (b == 6) )
- True
- Great!
- False
- Try again!
python-review25: What would the following print?:
a = 6
b = 10
print( a == 10 or b == 10 )
- True
- Great!
- False
- Try again! Notice that we did not ask a full question on the right side of the AND.
python-review26: What would the following print?:
a = 6
b = 10
print( a == 6 and 10 )
- True
- Great!
- False
- Try again!
python-review27: What would the following print?:
a = 6
b = 10
print( not a == 10 and b == 10 )
- True
- Try again!
- False
- Great!
python-review28: What would the following print?:
a = 6
b = 10
print( a == 10 or not b == 10 )
- True
- Great!
- False
- Try again!
python-review29: What would the following print?:
a = 6
b = 10
print( a == 6 and (not a == 10) )
- True
- Try again!
- False
- Great!
python-review30: What would the following print?:
a = 6
b = 10
print( not ( not a == 10 or not b == 10) )
2.9. Functions¶
Construct a function which returns the type of clothing you should wear, based on the parameter temperature. If the temperature is below -10, you will wear a parka and toque. If the temperature is between -10 and 0, wear a toque. If the temperature is greater than 0 but less than 10, wear a sweater. If the temperature is between 10 and 20, wear a t-shirt. If the temperature is greater than 20, wear shorts.
Note
The only thing you need to do for this question is to complete the function definition! You do not need to call the function, as that will be done automatically for you.
The parameter the_number
needs to be doubled, but only if the_number
is positive. Return the doubled value of the number that is passed in if the_number
is positive. If the_number
is negative, return -1. If the_number
is 0, return 0.
double_it_positive(5) → 10
double_it_positive(0) → 0
double_it_positive(-4) → -1
- Its value
- Value is the contents of the variable. Scope concerns where the variable is "known".
- The area in the code where a variable can be accessed.
- Great! Remember that a variable defined inside a function "disappears" after the function has finished executing.
- Its name
- The name of a variable is just an identifier or alias. Scope concerns where the variable is "known".
python-review33: What is a variable’s scope?
- A temporary variable that is only used inside a function
- Yes, a local variable is a temporary variable that is only known (only exists) in the function it is defined in.
- The same as a parameter
- While parameters may be considered local variables, functions may also define and use additional local variables.
- Another name for any variable
- Variables that are used outside a function are not local, but rather global variables.
python-review34: What is a local variable?
- Yes, and there is no reason not to.
- While there is no problem as far as Python is concerned, it is generally considered bad style because of the potential for the programmer to get confused.
- Yes, but it is considered bad form.
- It is generally considered bad style because of the potential for the programmer to get confused. If you must use global variables (also generally bad form) make sure they have unique names.
- No, it will cause an error.
- Python manages global and local scope separately and has clear rules for how to handle variables with the same name in different scopes, so this will not cause a Python error.
python-review35: Can you use the same name for a local variable as a global variable?
2.10. Drawing Images Practice¶
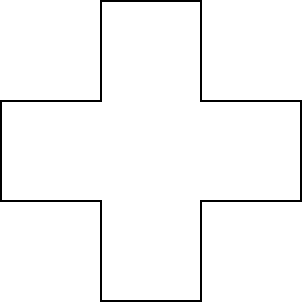
Construct a function that draws the image shown above:
Draw the following image. Be sure to define and use at least one function as part of your solution.
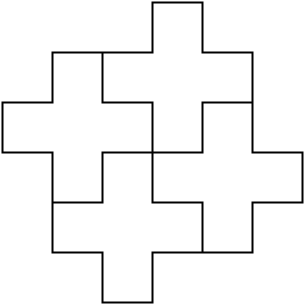
Once you have completed the shape above, try the following. Use the code you made above as a starting point.
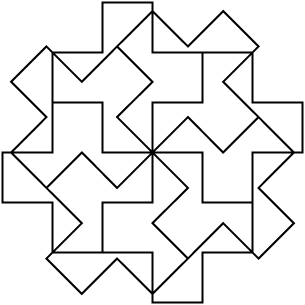
2.11. Image Manipulation with Nested Loops¶
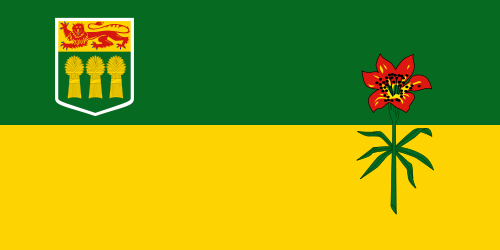
Construct a function that draws the negative of the image shown above. Note: due to technical limitations with this question, you need to use the x coordinate as your outer loop.
2.12. Strings¶
- t
- Index locations do not start with 1, they start with 0.
- h
- Yes, index locations start with 0.
- c
- sentence[-3] would return c, counting from right to left.
- Error, you cannot use the [ ] operator with a string.
- [ ] is the index operator
python-review40: What is printed by the following statements?
sentence = "python rocks"
print(sentence[3])
- tr
- Yes, indexing operator has precedence over concatenation.
- ps
- p is at location 0, not 2.
- nn
- n is at location 5, not 2.
- Error, you cannot use the [ ] operator with the + operator.
- [ ] operator returns a string that can be concatenated with another string.
python-review41: What is printed by the following statements?
sentence = "python rocks"
print(sentence[2] + sentence[-5])
- 11
- The blank counts as a character.
- 12
- Yes, there are 12 characters in the string.
python-review42: What is printed by the following statements?
sentence = "python rocks"
print(len(sentence))
- o
- Take a look at the index calculation again, len(sentence)-5.
- r
- Yes, len(sentence) is 12 and 12-5 is 7. Use 7 as index and remember to start counting with 0.
- s
- sentence is at index 11
- Error, len(sentence) is 12 and there is no index 12.
- You subtract 5 before using the index operator so it will work.
python-review43: What is printed by the following statements?
sentence = "python rocks"
print(sentence[len(sentence)-5])
- c
- Yes, 3 characters from the end.
- k
- Count backward 3 characters.
- s
- When expressed with a negative index the last character s is at index -1.
- Error, negative indices are illegal.
- Python does use negative indices to count backward from the end.
python-review44: What is printed by the following statements?
sentence = "python rocks"
print(sentence[-3])
- python
- That would be sentence[0:6].
- rocks
- That would be sentence[7:].
- hon r
- Yes, start with the character at index 3 and go up to but not include the character at index 8.
- Error, you cannot have two numbers inside the [ ].
- This is called slicing, not indexing. It requires a start and an end.
python-review45: What is printed by the following statements?
sentence = "python rocks"
print(sentence[3:8])
- 10
- Iteration by item will process once for each item in the sequence.
- 11
- The blank is part of the sequence.
- 12
- Yes, there are 12 characters, including the blank.
- Error, the for statement needs to use the range function.
- The for statement can iterate over a sequence item by item.
python-review46: How many times is the word HELLO printed by the following statements?
s = "python rocks"
for ch in s:
print("HELLO")
- 4
- Slice returns a sequence that can be iterated over.
- 5
- Yes, The blank is part of the sequence returned by slice
- 6
- Check the result of s[3:8]. It does not include the item at index 8.
- Error, the for statement cannot use slice.
- Slice returns a sequence.
python-review47: How many times is the word HELLO printed by the following statements?
s = "python rocks"
for ch in s[3:8]:
print("HELLO")
- ball
- Yes, the repeated concatenation will cause another_string to become the same as some_string.
- llab
- Look again at the *order* of the concatenation!
python-review48: What is printed by the following statements:
some_string = "ball"
another_string = ""
for item in some_string:
another_string = another_string + item
print(another_string)
- ball
- Look again at the *order* of the concatenation!
- llab
- Yes, the order is reversed due to the order of the concatenation.
python-review49: What is printed by the following statements:
some_string = "ball"
another_string = ""
for item in some_string:
another_string = item + another_string
print(another_string)
- 0
- There are definitely o and p characters.
- 2
- There are 2 o characters but what about p?
- 3
- Yes, add the number of o characters and the number of p characters.
python-review50: What is printed by the following statements?
s = "python rocks"
print(s.count("o") + s.count("p"))
Construct a block of code that correctly creates a function with a single parameter word that returns the even letters of the word, then calls the function and prints the result.
2.13. Lists¶
- 4
- len returns the actual number of items in the list, not the maximum index value.
- 5
- Yes, there are 5 items in this list.
python-review52: What is printed by the following statements?
a_list = [3, 67, "cat", 3.14, False]
print(len(a_list))
- 7
- Yes, there are 7 items in this list even though two of them happen to also be lists.
- 8
- len returns the number of top level items in the list. It does not count items in sublists.
python-review53: What is printed by the following statements?
a_list = [3, 67, "cat", [56, 57, "dog"], [ ], 3.14, False]
print(len(a_list))
- [ ]
- The empty list is at index 4.
- 3.14
- Yes, 3.14 is at index 5 since we start counting at 0 and sublists count as one item.
- False
- False is at index 6.
python-review54: What is printed by the following statements?
a_list = [3, 67, "cat", [56, 57, "dog"], [ ], 3.14, False]
print(a_list[5])
- 56
- Indexes start with 0, not 1.
- c
- Yes, the first character of the string at index 2 is c
- cat
- cat is the item at index 2 but then we index into it further.
- Error, you cannot have two index values unless you are using slicing.
- Using more than one index is fine. You read it from left to right.
python-review55: What is printed by the following statements?
a_list = [3, 67, "cat", [56, 57, "dog"], [ ], 3.14, False]
print(a_list[2][0])
- True
- Yes, 3.14 is an item in the list a_list.
- False
- There are 7 items in the list, 3.14 is one of them.
python-review56: What is printed by the following statements?
a_list = [3, 67, "cat", [56, 57, "dog"], [ ], 3.14, False]
print(3.14 in a_list)
- True
- in returns True for top level items only. 57 is in a sublist.
- False
- Yes, 57 is not a top level item in a_list. It is in a sublist.
python-review57: What is printed by the following statements?
a_list = [3, 67, "cat", [56, 57, "dog"], [ ], 3.14, False]
print(57 in a_list)
- [ [ ], 3.14, False]
- Yes, the slice starts at index 4 and goes up to and including the last item.
- [ [ ], 3.14]
- By leaving out the upper bound on the slice, we go up to and including the last item.
- [ [56, 57, "dog"], [ ], 3.14, False]
- Index values start at 0.
python-review58: What is printed by the following statements?
a_list = [3, 67, "cat", [56, 57, "dog"], [ ], 3.14, False]
print(a_list[4:])
- [4, 2, 8, 6, 5, False, True]
- True was added first, then False was added last.
- [4, 2, 8, 6, 5, True, False]
- Yes, each item is added to the end of the list.
- [True, False, 4, 2, 8, 6, 5]
- append adds at the end, not the beginning.
python-review59: What is printed by the following statements?
a_list = [4, 2, 8, 6, 5]
a_list.append(True)
a_list.append(False)
print(a_list)
- [4, 8, 6]
- pop(2) removes the item at index 2, not the 2 itself.
- [2, 6, 5]
- pop() removes the last item, not the first.
- [4, 2, 6]
- Yes, first the 8 was removed, then the last item, which was 5.
python-review60: What is printed by the following statements?
a_list = [4, 2, 8, 6, 5]
temp = a_list.pop(2)
temp = a_list.pop()
print(a_list)
- [2, 8, 6, 5]
- a_list is now the value that was returned from pop(0).
- [4, 2, 8, 6, 5]
- pop(0) changes the list by removing the first item.
- 4
- Yes, first the 4 was removed from the list, then returned and assigned to a_list. The list is lost.
- None
- pop(0) returns the first item in the list so a_list has now been changed.
python-review61: What is printed by the following statements?
a_list = [4, 2, 8, 6, 5]
a_list = a_list.pop(0)
print(a_list)
Note
The only thing you need to do for this question is to complete the function definition! You do not need to call the function, as that will be done automatically for you.
Write a function to count how many odd numbers are in a list.
Examples:
count_odds([1,3,5,7,9]) → 5
count_odds([1,2,3,4,5]) → 3
count_odds([2,4,6,8,10]) → 0
2.14. Number Guessing Game¶
Implement a number guessing game in Python that does the following:
generate a random number from 1 to 100 and stores it in a variable
repeat the following until the user guesses the number:
get the user to guess the number
tell the user if the number is too high or too low
congratulate the user when they guess the correct number with a message such as “Way to go! You guessed the right number in 9 tries!”