2. Writing Fruitful Functions Practice¶
Quick Overview of Day
Warmup drawing problem. WDTPD questions about functions. Students practice writing fruitful functions.
CS20-CP1 Apply various problem-solving strategies to solve programming problems throughout Computer Science 20.
CS20-FP1 Utilize different data types, including integer, floating point, Boolean and string, to solve programming problems.
CS20-FP2 Investigate how control structures affect program flow.
CS20-FP3 Construct and utilize functions to create reusable pieces of code.
2.1. Warmup Problem¶
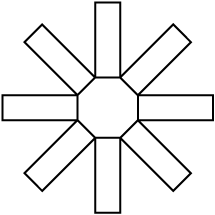
Draw the image above using the Python turtle module. You must define a function as part of your solution!
2.2. What Does This Program Do?¶
Remember that variables created inside of a function have local scope (can only be used inside that function), whereas variables created outside of a function have global scope (can be accessed from anywhere).
Note
Your teacher may choose to use the following examples as a class activity, by displaying the examples, and having you take a guess as to what you think each will do before running the code.
What will the following programs output? Why?
2.3. Practice Problems¶
Try the following practice problems to be sure you understand how to create fruitful functions. Your functions have to return the correct value – using print()
will not work. When you run your code for these questions, your code will automatically be checked with a number of test cases to see if your function works in all situations. You will be able to see any situations in which your function does not provide the correct answer.
Note
The only thing you need to do for the following is to complete the function definition! You do not need to call the function, as that will be done automatically for you.
2.3.1. Area of rectangle¶
The parameters length
and width
represent the lengths of the sides of a rectangle. Calculate the area of the rectangle with the given values, and return the result.
Examples:
rectangle_area(5, 10) → 50
rectangle_area(1, 10) → 10
rectangle_area(2, 6) → 12
2.3.2. Letter Grade¶
Write a function that returns the letter grade, given an exam mark as the parameter. The grading scheme is:
Letter Grade |
Interval |
---|---|
A |
>= 90 |
B |
[80, 90) |
C |
[70, 80) |
D |
[60, 70) |
F |
< 60 |
The square and round brackets denote closed and open intervals. A closed interval includes the number, and open interval excludes it. So 79.99999 gets grade C, but 80 gets grade B.
Examples:
letter_grade(83) → "B"
letter_grade(73) → "C"
letter_grade(80) → "B"
2.3.3. Find the Smallest¶
The function find_min(a, b, c) will take three numbers as parameters and return the smallest value. If more than one number is tied for the smallest, still return that smallest number. Note that you cannot use the min
function in this solution.
Examples:
find_min(4, 7, 5) → 4
find_min(4, 5, 5) → 4
find_min(4, -7, 5) → -7
2.3.4. Is Even¶
The function is_even(number) will return True
if the number passed in is even, and False
if it is odd. Hint: You might want to look back at the Math Operators list.
Examples:
is_even(4) → True
is_even(-4) → True
is_even(5) → False
2.3.5. Leap Year¶
A year is a leap year if it is divisible by 4 unless it is a century that is not divisible by 400. Write a function that takes a year as a parameter and returns True if the year is a leap year, False otherwise. The following pseudocode determines whether a year is a leap year or a common year in the Gregorian calendar (from Wikipedia):
if (year is not divisible by 4) then (it is a common year)else if (year is not divisible by 100) then (it is a leap year)else if (year is not divisible by 400) then (it is a common year)else (it is a leap year)
Examples:
leap_year(2001) → False
leap_year(2020) → True
leap_year(1900) → False
2.3.6. Using Your Is Even Function¶
Write a program that continues to take in a number from the user until the number given is NOT even. For example, the user might enter 4, 10, 42, 5. The program would only stop when the non-even number 5 is entered. You need to use the is_even
function you defined above.